Ruby on Rails
This tutorial will show you how to use the Lokalise preprocessing and postprocessing feature to manage uploaded and downloaded translations in your Rails app.
In this tutorial you'll learn how to...
Implement custom processors for Lokalise using Ruby on Rails.
Prerequisites
This guide assumes that you have already created a Lokalise project (if not, learn how to create a new project in this guide).
If you want to follow this guide locally on your computer, you need to have the following software installed:
What we are going to build
We are going to create a simple application that will process translations that you'll import and export on Lokalise. The app will then process translations and remove any banned words.
Preparing the app
Let's start with preparing a Rails app. Create it by running:
rails new CustomProcessors
Next let's create two POST routes to perform preprocessing and postprocessing inside the config/routes.rb
file:
post '/preprocess', to: 'processors#preprocess'
post '/postprocess', to: 'processors#postprocess'
Also create a new controller inside the app/controllers/processors_controller.rb
file:
class ProcessorsController < ApplicationController
skip_before_action :verify_authenticity_token, only: %i[preprocess postprocess]
def preprocess
end
def postprocess
end
end
We have to skip authenticity token verification as translations will be sent by a third-party resource.
Performing preprocessing
So, now let's see how to perform translations preprocessing. For example, suppose we want to iterate over the uploaded translations and remove a "BANNED" word from them.
To achieve that, simply tweak the preprocess
action:
def preprocess
payload = params
payload[:collection][:keys].each do |key|
key[:translations].map! do |trans|
trans[:translation].gsub!(/BANNED/, '')
trans
end
end
render json: payload
end
Here we are iterating over translations and use gsub!
to replaced "BANNED" with an empty string.
Also note that we must respond with a JSON payload preserving the original structure otherwise the import will fail.
Enabling preprocessor
Now that the preprocessor is ready, we have to enable it on Lokalise. To achieve that, open your Lokalise project, proceed to Apps, find Custom processor in the list and click on it. Then click Install and configure this app by entering a preprocess URL:
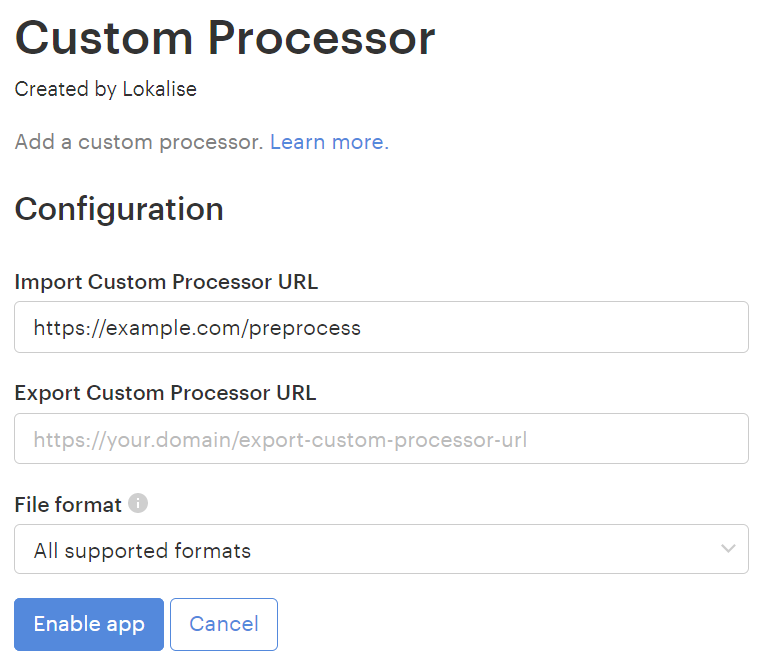
By default preprocessor will be run for every uploaded translation file but you can narrow the scope by choosing one of the file formats from the corresponding dropdown.
Once you are ready, click Enable app. Great job!
Postprocessing translations
Now let's perform a very similar thing and remove "BANNED" words from the downloaded translations.
To achieve that, tweak the postprocess
action:
def postprocess
payload = params
payload[:collection][:keys].each do |key|
key[:translations].map! do |trans|
trans[:translation].gsub!(/BANNED/, '')
trans
end
end
render json: payload
end
This is it!
Enabling postprocessing
Now that the app is finalized, we have to enable postprocessing on Lokalise. To achieve that, open your Lokalise project, proceed to Apps, find Custom processor in the list and click on it. Then click Install and configure this app by entering a postprocess URL:
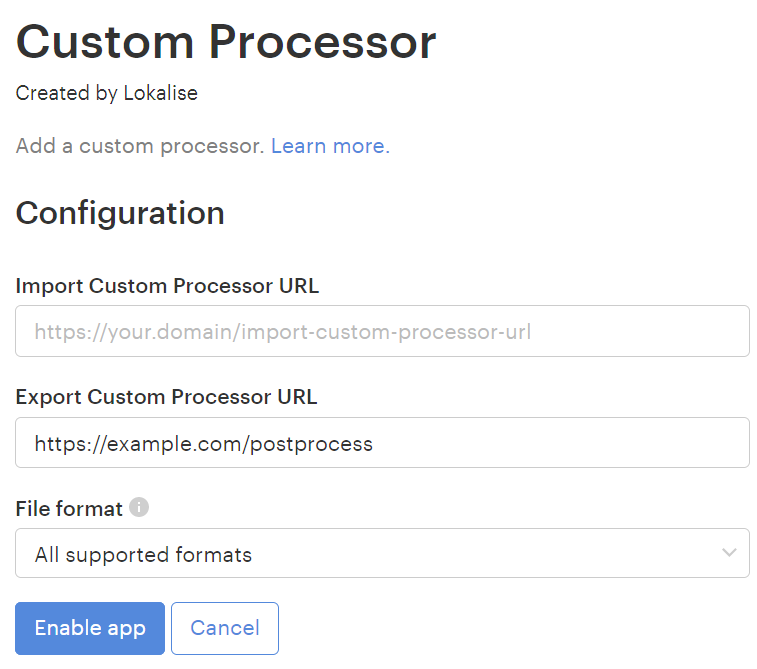
By default postprocessor will be run for every uploaded translation file but you can narrow the scope by choosing one of the file formats from the corresponding dropdown.
Once you are ready, click Enable app. Great job!
Updated almost 3 years ago